Python - 面向对象
- Object-Oriented Fundamentals
- Requirements
- Use Cases and User Stories
- Domain Modeling
- Class Diagrams
- Inheritance and Composition
- Software Development
Object-Oriented Fundamentals
- What’s object?
- 真实世界中的对象
- 对象有各种attributes属性 (名词)
- 对象有各种behaviors方法 (动词)
- What’s class?
- 将现实世界中的对象抽象
- code-template for creating program objects
- Four foundation ideas
- Abstraction抽象
- Polymorphism多态
- use the same interface for methods on different types of objects
- overriding (重写)
- method overloading (重载)
- 实现方法,方法名相同,但是参数不同
- Inheritance继承
- python 可以继承多个父类
- Encapsulation封装
- Three steps for OO
- Analysis
- Understand your problems
- Design
- Plan your solutions
- Programming
- Build it
- Analysis
- UML
- Steps
-
- Class Diagram
-
Requirements
- Functional requirements:
- What must do?
- Non-Functional requirements:
- What should be?
- FURPS
- Functionality
- Usability
- Reliablility
- Performance
- Supportability
Use Cases and User Stories
- Use Cases
- title
- actor
- how to identiy?
- 交互对象
- primary actor is user for the application initialtion
- how to identiy?
- success scenario
- how to identiy
- 确认用户目标
- focus on the typical situation that would occur
- active voice, easy to read, short and concise
- how to identiy
- Diagram use case
- make actor connect with scenario
- Use Stories
-
Domain Modeling
- Indentify objects
- 通过分析 use cases 中的名词
- Indentiy class relationships
- 将object 用动词关联
- Indentiy class responsibilities
- 通过分析 use cases 中的动词
- CRC cards
- class, responsibility and collaboration
Class Diagrams
- UML diagram for class
- Convert class diagram into code
- Instantiation
- Constructor
- 初始化attribute
- 通过overloading, 可以有多个构造函数
-
- Destructoer
- Finalizer
- Static attribues and methods
-
- classname.静态属性/方法,非静态对象,使用实例名称,eg:Instantiation.name
-
- static in UML
- 使用下划线
-
-
Inheritance and Composition
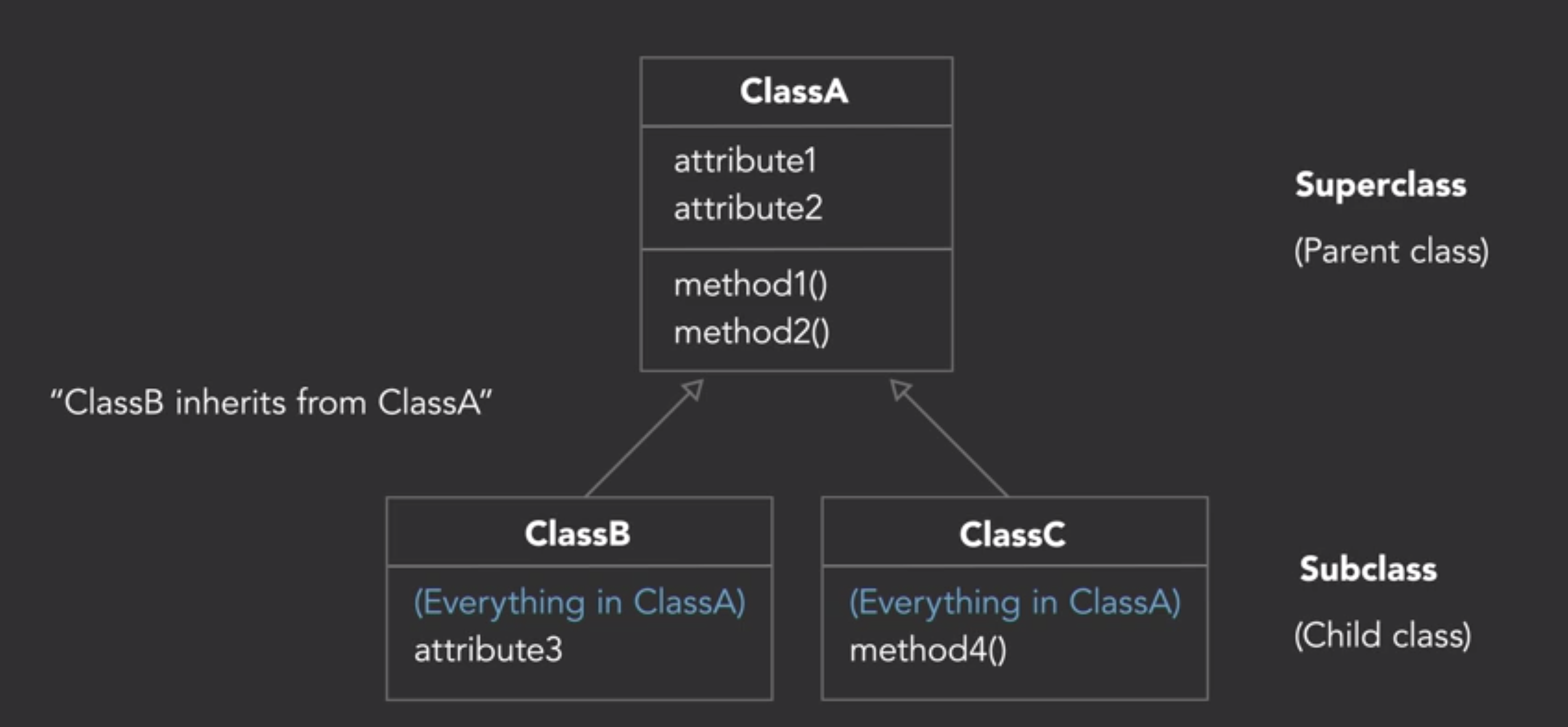
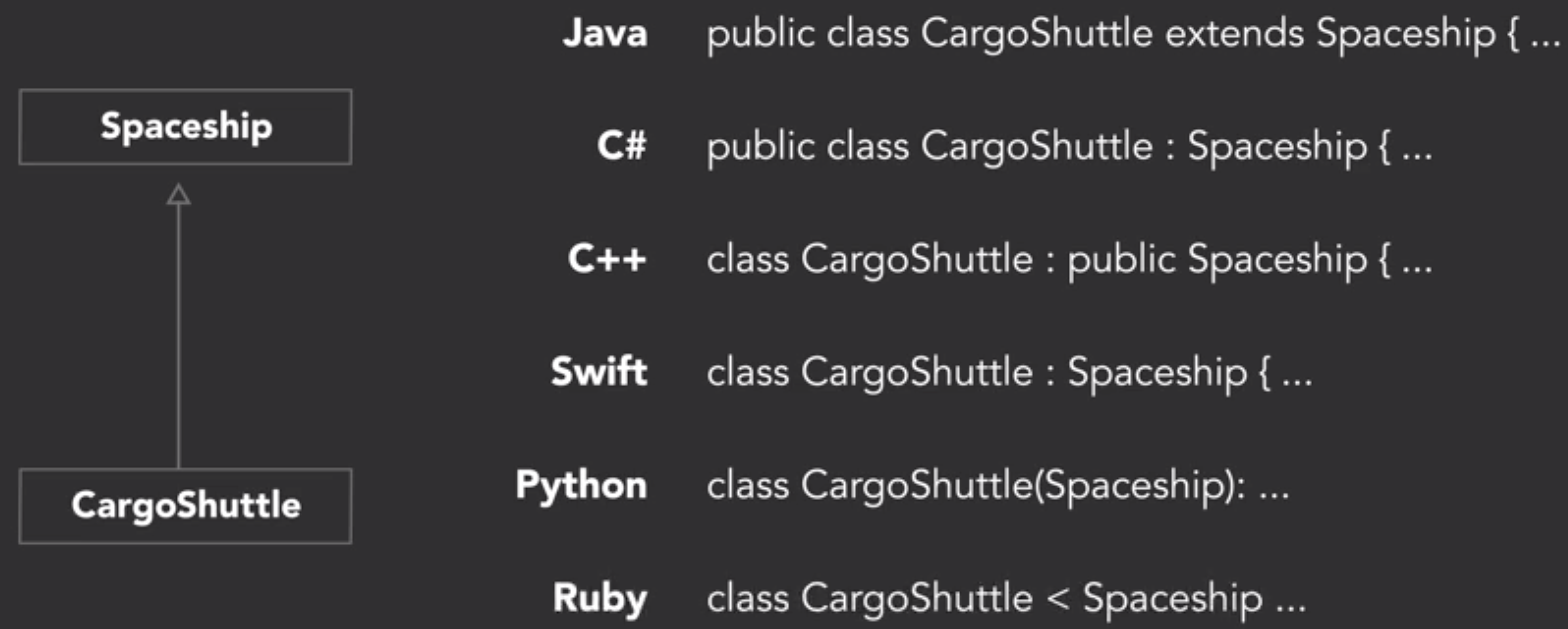
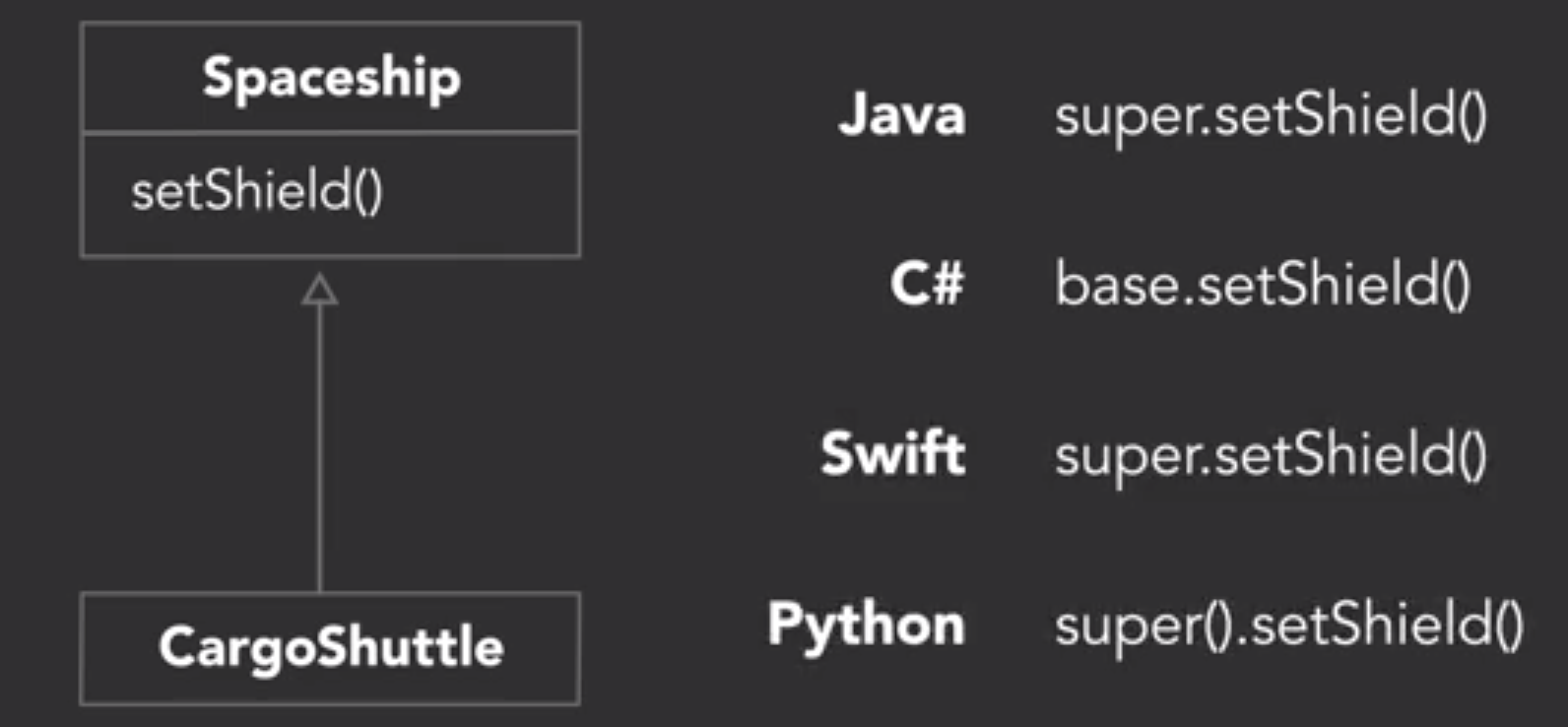
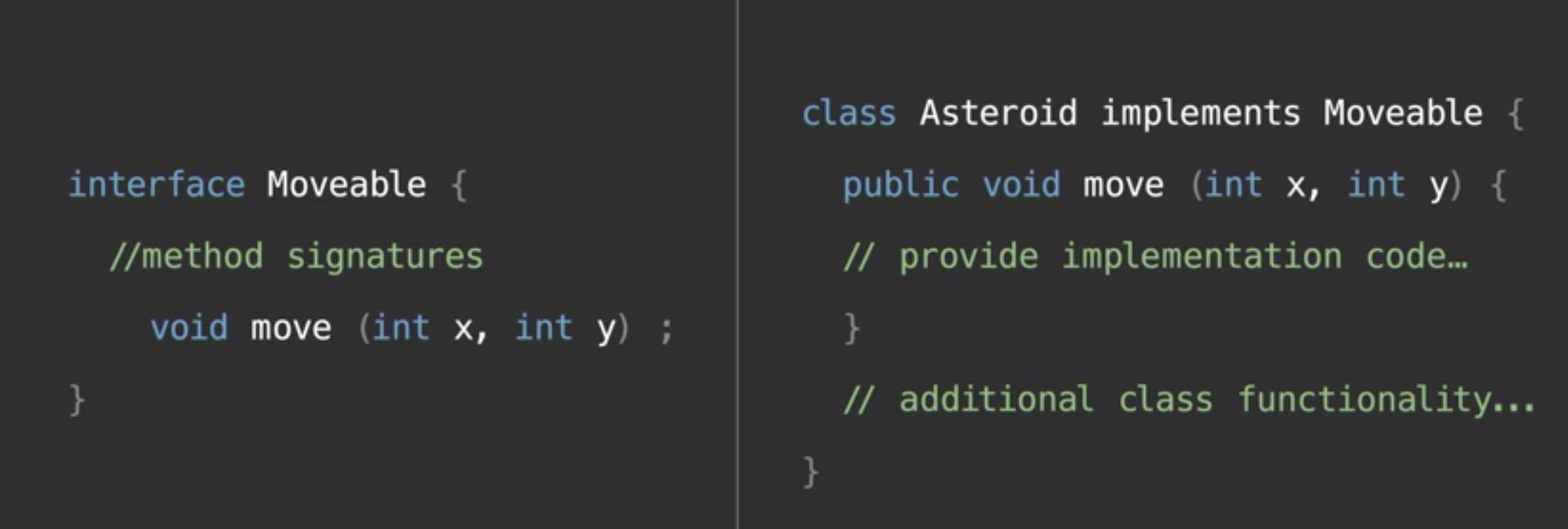
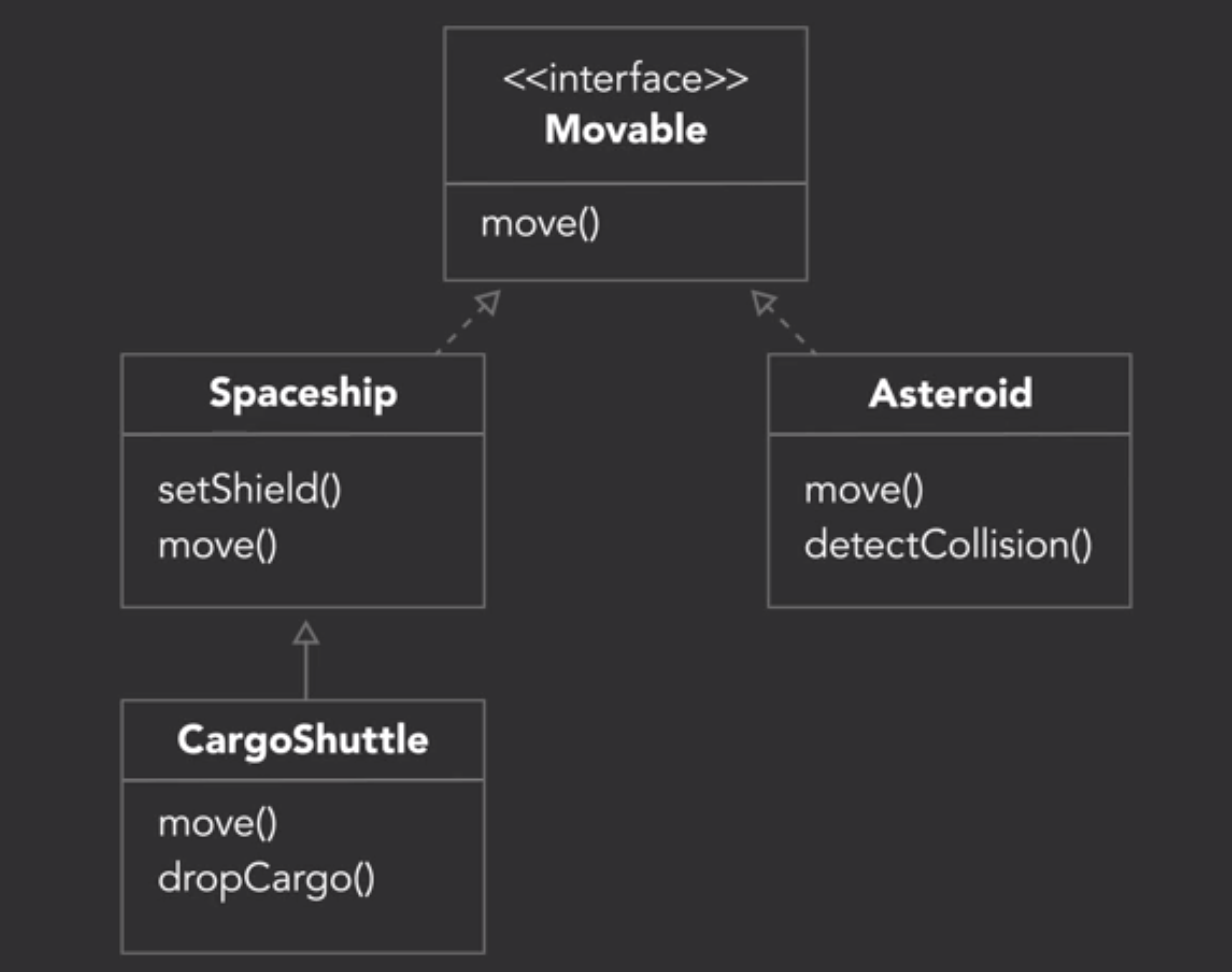
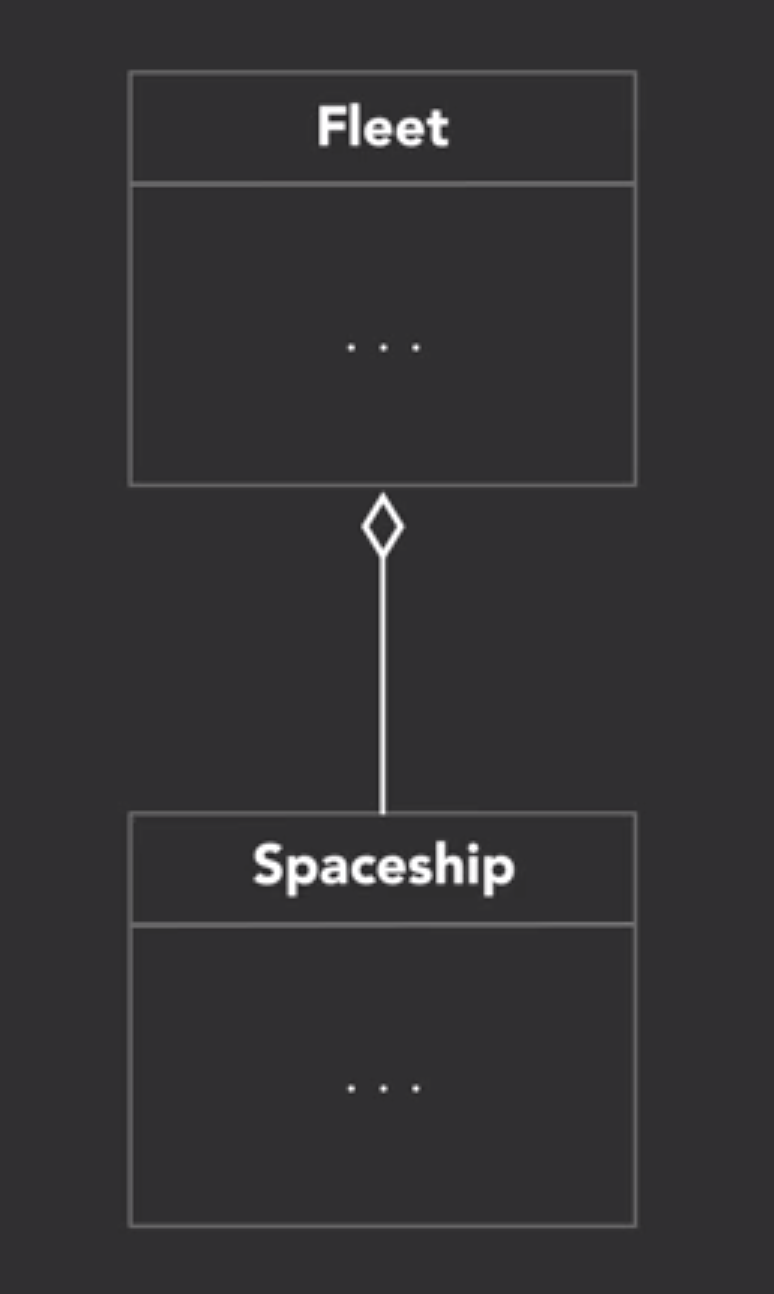
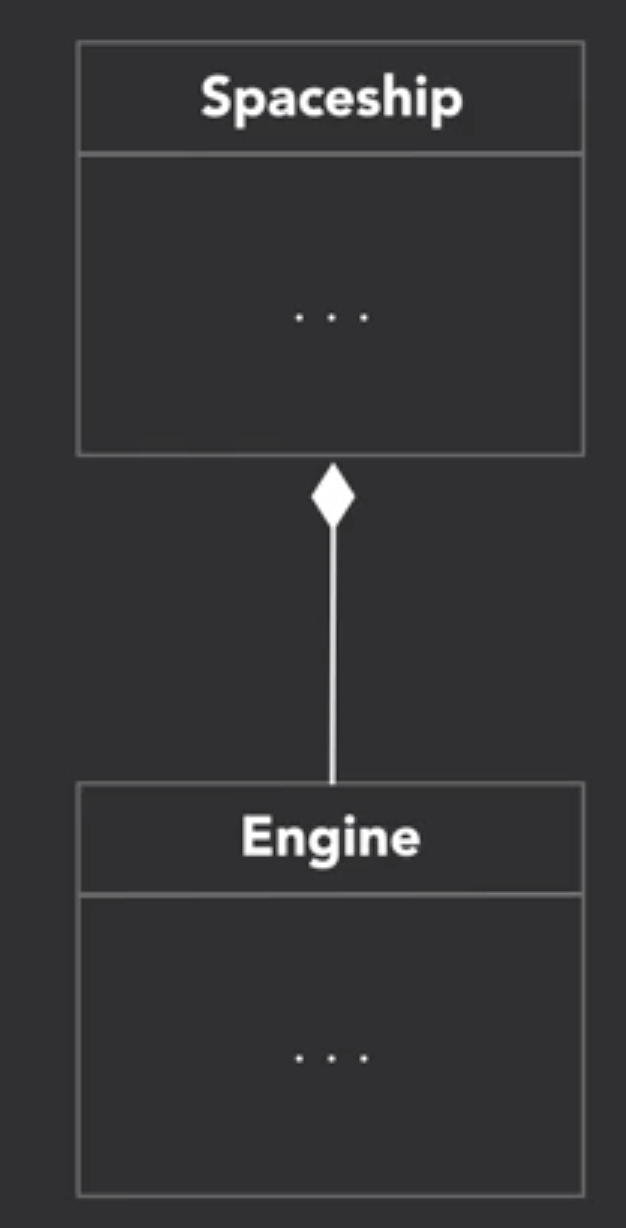
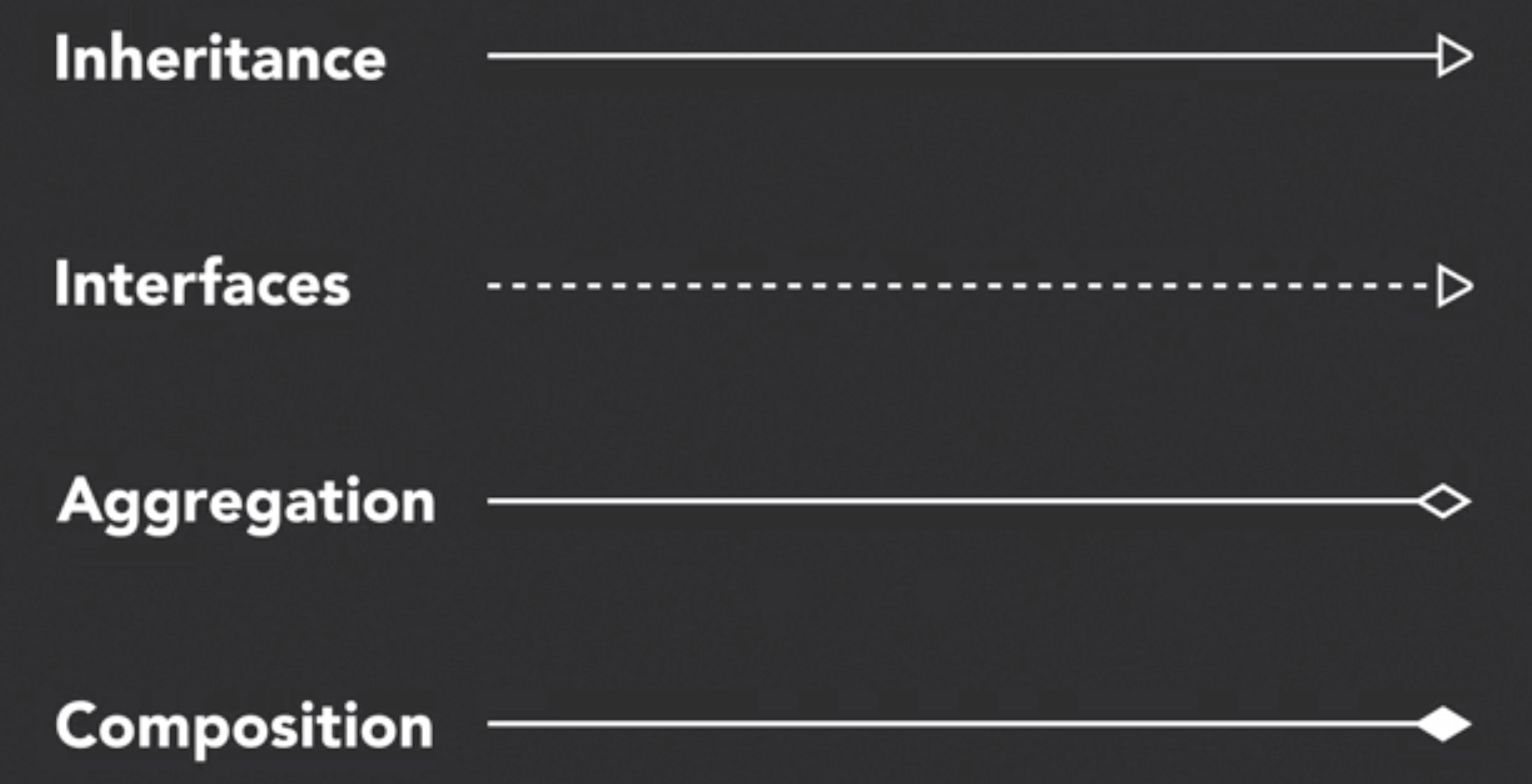
Software Development
- 面向对象语言对比
- Dev principle
- Single responsibility principle
- a class should have only a single reponsibility
- DRY
- don’t repeat yourself
- YAGNI
- you ain’t gonna need it
- Code Smell
- sniff test
- Single responsibility principle
- Software testing
- Design patterns
- factory method pattern
- memento pattern
-
- 参考书
- “Design Patterns” by Gang of Four book
- “Head First Design Patterns”
- factory method pattern